In the world of web development, Markdown has become a popular choice for writing content due to its simplicity and readability. However, serving Markdown files directly in a web application requires rendering them into HTML.
In this blog post, we will explore how to render a Markdown file using Flask and display it as plain HTML in the web browser.
So whether you're building a personal blog, a documentation site, or just looking to integrate Markdown into your web next Flask project, this guide will walk you through the process step by step.
Let's dive in!
But first... What is Markdown?
As mentioned on the IBM Docs, "Markdown is an easy-to-use markup language that is used with plain text to add formatting elements (headings, bulleted lists, URLs) to plain text without the use of a formal text editor or the use of HTML tags. Markdown is device agnostic and displays the writing format consistently across device types.".
Markdown files have the .md extension and they're written using a specific syntax.
Rendering a Markdown file (.md) in Flask
NOTE: For this tutorial, I'll assume that you know your way around Python and Flask, and I'll focus mainly on the actual script code.
In this tutorial, I'll show you how to render the content of the file temp.md and display it in the browser using Flask. For this I'll work with an extension called Flask-Markdown.
Folder structure
├── templates
│ └── index.html
├── app.py
├── requirements.txt
└── temp.md
This is the folder structure that I've used:
templates folder is where I'll keep my templates, in this case is just the index.html;
app.py is the actual Python script;
requirements.txt is the pip requirements to install in order for the script to run;
temp.md is the Markdown file to render;
temp.md
## Markdown to HTML in Flask - Test document
This is a test document.
app.py
from flask import Flask, render_template
from flaskext.markdown import Markdown
# Initialize the Flask application
app = Flask(__name__)
# Initialize Flask-Markdown extension
Markdown(app)
@app.route('/')
def index():
# Read the Markdown file content
with open("temp.md") as md_file:
data=md_file.read()
# Render the template with the Markdown content
return render_template('index.html', md_content=data)
if__name__=='__main__':
app.run(debug=True)
index.html
...
<body>
<div class="main-post">
{{ md_content|markdown }}
</div>
</body>
...
Result:
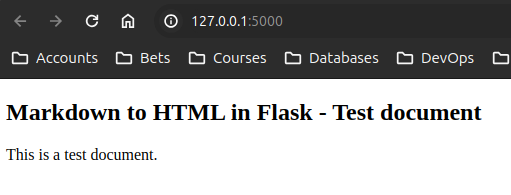
And this is it! This is how you can render Markdown as HTML while using Flask. You can find the source code here and if you have any questions, feel free to reach me out through my social media. See ya!